Arrays
Arrays
Difficulty : Beginner-Intermediate
This tutorial assumes you have a basic understanding of coding in Processing or p5, and are comfortable using variables, if statements and for loops.
Introduction : The What and the Why
In order to understand why So far we've learnt that we can use variables to name data in our code, as so:
var myVariable = 200;
console.log(myVariable);
And we know that we can use these variable in our sketch. In the following example I create variables X and Y, and use them to as the x and y coordinates in an ellipse function
var x = 100;
var y = 100;
function setup() {
createCanvas(400, 400);
}
function draw() {
background(220);
ellipse(x, y, 20,20);
}
Now what if we wanted to have a lot of circles, like in the image below?
You might be thinking of doing something like this, with seperate variables for every ellipse:
var x1 = 20;
var y1 = 100;
var x2 = 213;
var y2 = 209;
var x3 = 100;
var y3 = 100;
var x4 = 100;
var y4 = 100;
And you'd be right! This is a perfectly valid approach. However, imagine if we had hundreds of circles, we'd need hundreds of variables as well. Things would start getting messy quickly and we'd have to make sure we were keeping track of everything individually. Going further, say we wanted to manipulate all of our circles in a similar way (for example, move them all left) we would end up with lots and lots of lines of really similar code. Wouldn't it be good if we could handle all this data at once?
Well... WE CAN! This is what arrays are perfect for. An erray is essentially just like other variables, only instead of storing one piece of information they store many. Arrays work almost exactly the same in both p5 and Processing but there are a few key differences. We will be using p5 in this tutorial, but I have included a section at the end going over these differences and how to use arrays in Processing.
Arrays in p5
The easiest way to create an array in p5 is a s follows:
var myArray = [ 20, 213, 100, 150, 342];
We declare the variable myArray just as we would any other variable, but rather than providing just one value after the '=' we've included several. These values must be within square brackets '[ ]' and must be seperated by a comma. Now, whenever we use myArray in our code we are actually referring to the whole list of numbers: 20, 213, 100, 140 342.
Ok great, but how can we use the information we've stored in our array? Once again we need to use the square brackets! Write the name of the array followed by square brackets containing a number corresponding to the elelemnt of the list you want to access. Copy the following code into a new p5 sketch, or click on the link to open the example in the editor.
var myArray = [20, 213, 100, 150, 342];
console.log( myArray[1] );
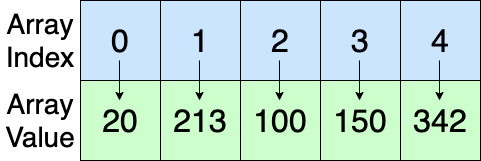